Few developers that have been involved in any form of web api development of the past few years will not be aware of the OSS framework api tool, Swagger. At whichever stage of its offering you opt to join, one of the most popular tools is its auto-generated document solution.
If you don’t have a public api to use and don’t want to engage with the online editor itself, it’s good to know that you can create your own swagger UI from within your dotnet core code with just a few simple steps. I’m using a blank asp.net core 2.1 web api project. Note that all the code can be found on Github at
https://github.com/agiletea/poc-api-examples
Simple User Api
Given a simple User Controller class with some basic verbs:
[Route("api/[controller]")]
[ApiController]
public class UserController : ControllerBase
{
// GET: api/User
/// <summary>
/// List all users from the database
/// </summary>
/// <returns></returns>
[HttpGet]
public IEnumerable<User> Get()
{
return new []
{
new User{ Id = 1, FirstName = "Ben", LastName="Jones"},
new User{ Id = 2, FirstName = "Rakhee", LastName="Smith"},
new User{ Id = 3, FirstName = "Lola", LastName="Jones"},
new User{ Id = 4, FirstName = "Amelie", LastName="Smith"}
};
}
// GET: api/User/5
[HttpGet("{id}", Name = "GetById")]
public string Get(int id)
{
return "value";
}
// POST: api/User
[HttpPost]
public void Post([FromBody] string value)
{
}
// PUT: api/User/5
[HttpPut("{id}")]
public void Put(int id, [FromBody] string value)
{
}
// DELETE: api/ApiWithActions/5
[HttpDelete("{id}")]
public void Delete(int id)
{
}
}
Swashbuckle
This is a nuget package that targets .net Standard 2.0 and brings all the necessary swagger tools into your solution for documenting your asp.net core APIs. Search for Swashbuckle.AspNetCore in your package manager to install.
The next step is to add the swagger generation service (in ConfigureServices method of Startup.cs):
services.AddSwaggerGen(opt =>
{
opt.SwaggerDoc("v1", new Info
{
Version = "1.0",
Title = "Agile Tea User Api Service",
Description = "Setting up swagger ina sp.net dotnet core....."
});
});
Once this is done, you need to configure the app to use swagger and generate to UI (in the Configure method of Startup.cs):
app.UseSwagger();
app.UseSwaggerUI(opt =>
{
opt.SwaggerEndpoint("/swagger/v1/swagger.json", "User Core Api");
});
Xml Comments
There’s a neat trick to enabling the auto generation of your xml comments in this approach as well. First you need to generate the xml documentation on build by enabling the flag in the build options of the project and providing a path:
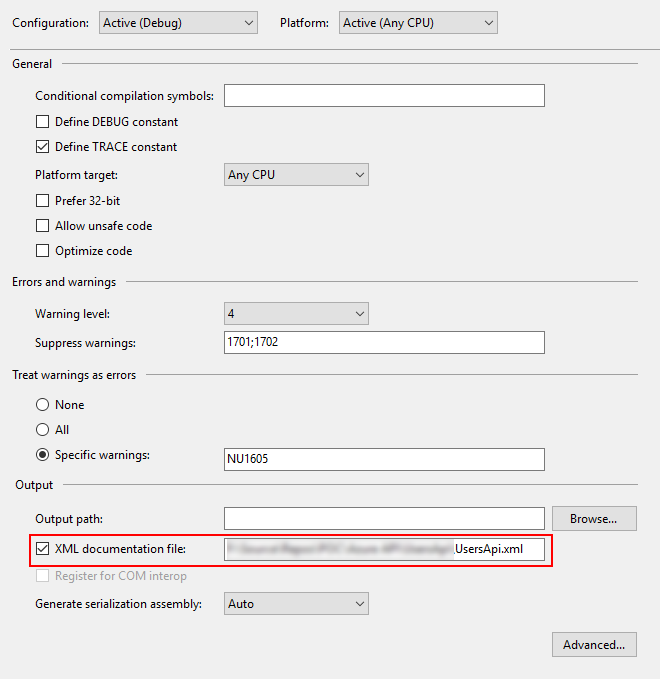
Then add the following line of code to the Configure Services section (assuming your xml file is generated in the base directory of your project):
opt.IncludeXmlComments($"{System.AppDomain.CurrentDomain.BaseDirectory}UsersApi.xml");
I find it handy to set by virtual path in my debug settings to ‘swagger’ so that the browser navigates straight to it on launch. When you launch you should then be presented with a screen similar to the following:
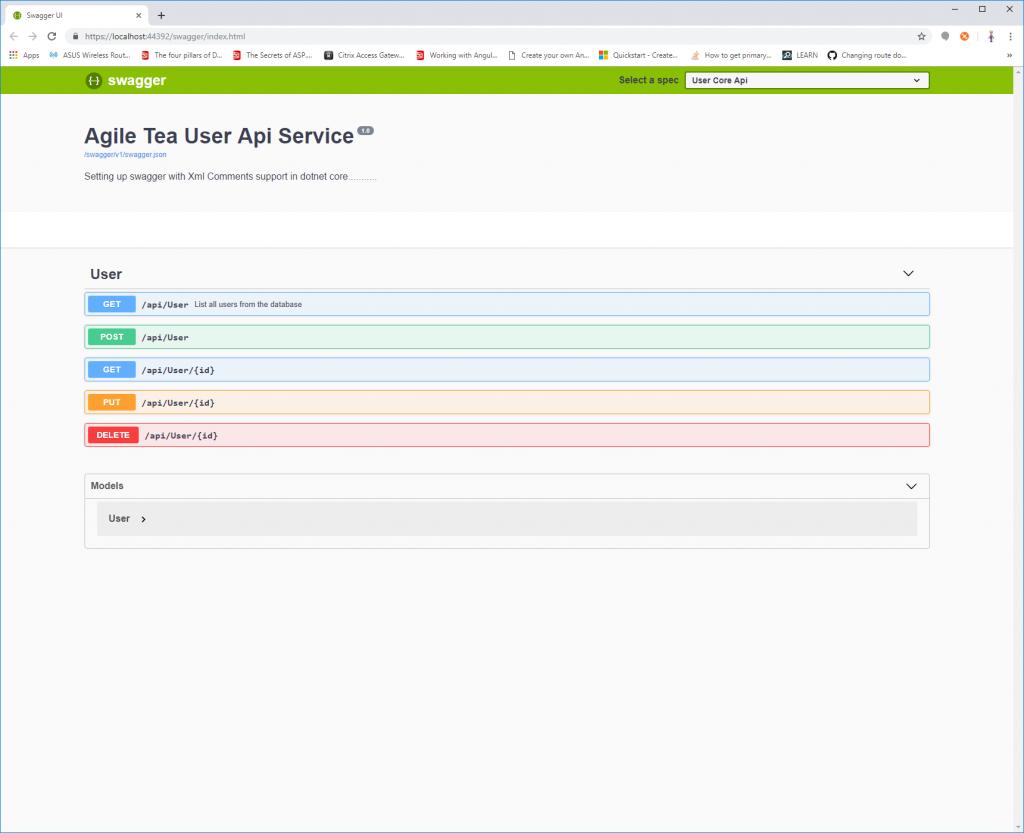
Trying it out demonstrates how valuable a tool this can be not just in terms of other developers understanding how to consume such an api, but less technical members of the project can at least gain an understanding of what the system can provide:
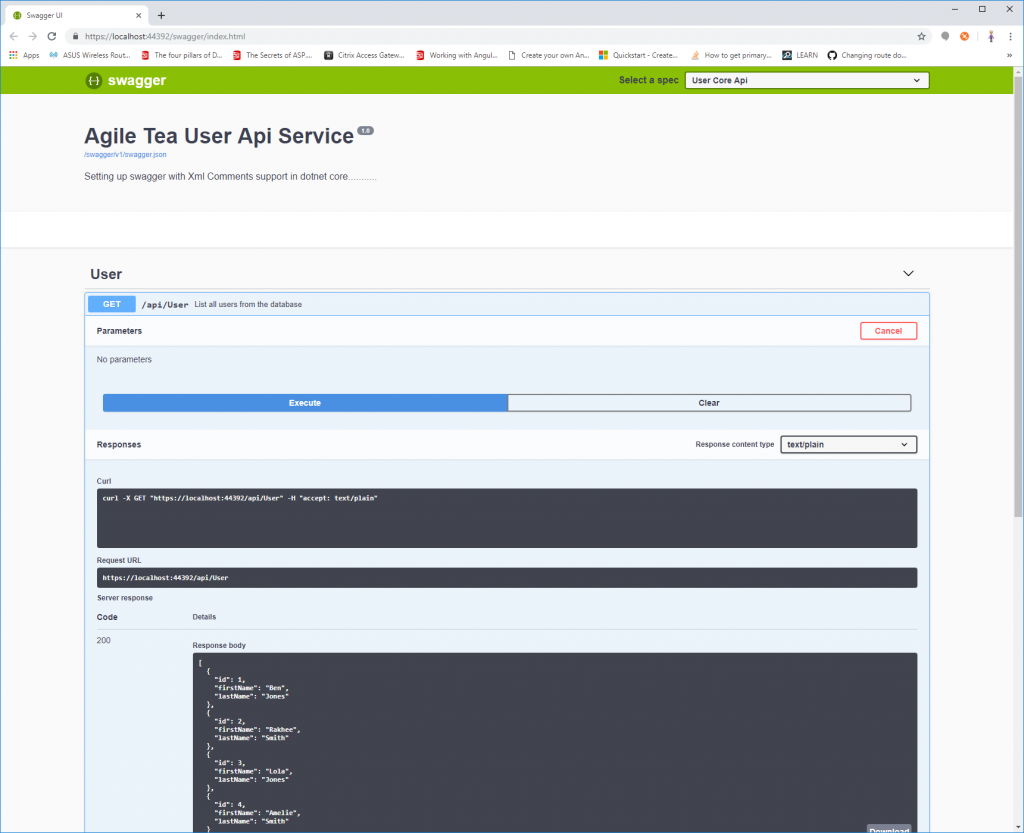
I’d estimate all of this took less than 15 minutes (not including the api prep) so it’s a great return on minimal effort.
0 Comments